MCU 8051 IDE
Skočit na navigaci
Skočit na vyhledávání
![]() ![]() | |
popis: | Vývoj aplikace pro 8051 v asm nebo C |
vývojář | Martin Ošmera |
aktuální verze | 1.4.9 |
OS | Unix-like, Microsoft Windows, freeBSD |
typ softwaru | aplikační |
licence | GNU General Public License |
web | wiki Download |
Založení nového projektu
- nastavte si přístup na svůj domovský disk (v učebně VYT5 je problematické použití písmena H) vhodnější nastavení je např.: net use Z: \\obelix\students\jmeno
- Project name: vyplňte název projektu
- Project directory: vypište Z:/MIT3r
- Vše ostatní nechte
-
úvodní obrazovka
-
založení nového projektu
-
cesta k adresáři Z:/MIT3r
Simulace programu
Example
Pro vývoj aplikace v C nutno nainstalovat SDCC - Small Device C Compiler
Write to Port
start:
mov A,#01010101b
mov P3,A
acall delay
mov A,#10101010b
mov P3,A
acall delay
sjmp start
delay: mov R0,#01h ; 108 ms
skok1: mov R1,#100
skok2: mov R2,#250
skok: DJNZ R2,skok
DJNZ R1,skok2
DJNZ R0,skok1
ret
end
|
#include <at89x51.h>
void _delay_ms(unsigned int msec) //for 11.0592Mhz crystal
{
unsigned int i,j;
for(i=0;i<msec;i++)
{
for(j=0;j<110;j++);
}
}
void main ()
{
while(1)
{
P3 = 0x55;
_delay_ms(100); // 100 ms
P3 = 0xAA;
_delay_ms(100);
}
}
|
Read from Ports
org 0 ;pseudoinstrukce umistujici program do pameti programu pocitace adresou 000h
start:
mov A,#P1
mov P3,A
sjmp start
Runing Light
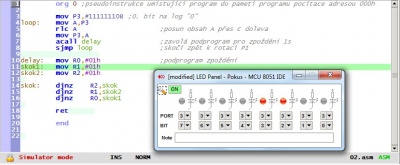
org 0 ;pseudoinstrukce umistujici program od 00h
mov P3,#11111110B ;
loop: mov A,P3
rlc A ;
mov P3,A
acall delay ;
sjmp loop ;
delay: mov R0,#1 ;
skok1: mov R1,#226
skok2: mov R2,#255
skok: djnz R2,skok
djnz R1,skok2
djnz R0,skok1
ret
end
Runing Light C
#include <at89x51.h>
#define LED P2_3
void main()
{
int i;
while(1)
{
if (LED)
{
LED = 0;
}
else
{
LED = 1;
}
{
for(i=0;i<10000;i++);
}
}
}
|
#include <at89x51.h>
#include <delay.h>
void main()
{
while (1)
{
P1=0xff;
_delay_ms(50); // 50 ms
P1=0x00;
_delay_ms(50); // 50 ms
}
}
/* file delay.h contains:
unsigned int i,j;
void _delay_ms(unsigned int msec) // crystal 10 059 kHz
{
for(i=0;i<msec;i++)
for(j=0;j<110;j++);
}
|
Blink using TIMER
#include <at89x51.h>
void main()
{
TMOD=0x10;
TR1=1;
for(;;)
{
P1_0=!P1_0;
TH1=0xFE;
TL1=0x0C;
while(TF1==0);
TF1=0;
}
}
|
7 segment
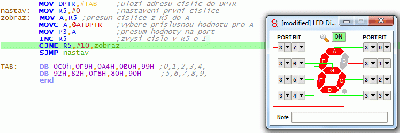
MOV DPTR,#TAB
nastav: MOV R5,#0
zobraz: MOV A,R5
MOVC A,@A+DPTR
MOV P3,A
INC R5
CJNE R5,#10,zobraz
SJMP nastav
TAB: DB 0C0h,0F9H,0A4H,0B0H,99H ;0,1,2,3,4,
DB 92H,82H,0F8H,80H,90H ;5,6,7,8,9,
end
7 segment v2
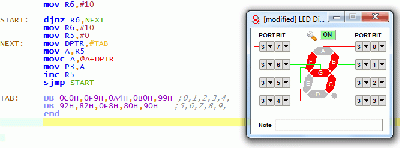
mov R6,#10
START: djnz R6,NEXT
mov R6,#10
mov R5,#0
NEXT: mov DPTR,#TAB
mov A,R5
movc A,@A+DPTR
mov P3,A
inc R5
sjmp START
TAB: DB 0C0H,0F9H,0A4H,0B0H,99H ;0,1,2,3,4,
DB 92H,82H,0F8H,80H,90H ;5,6,7,8,9,
end
7 segment - C
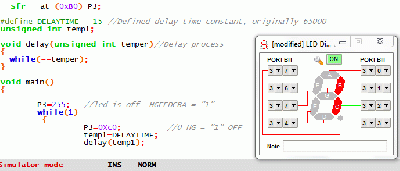
__sfr __at (0xB0) P3;
#define DELAYTIME 15 //Defined delay time constant, originally 65000
unsigned int temp1;
void delay(unsigned int temp)//Delay process
{
while(--temp);
}
void main()
{
P3=255; //led is off HGFEDCBA = "1"
while(1)
{
P3=0Xc0; //0 HG = "1" OFF
temp1=DELAYTIME;
delay(temp1);
P3=0XF9; //1 BC = "0" = ON
temp1=DELAYTIME;
delay(temp1);
}
}
7 segment - C (tab)
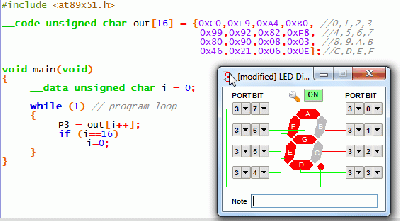
#include <at89x51.h>
__code unsigned char out[16] = {0xC0,0xF9,0xA4,0xB0, //0,1,2,3
0x99,0x92,0x82,0xF8, //4,5,6,7
0x80,0x90,0x08,0x03, //8.9.A.B
0x46,0x21,0x06,0x0E};//C,D,E,F
void main(void)
{
__data unsigned char i = 0;
while (1) // program loop
{
P3 = out[i++];
if (i==16)
i=0;
}
}
Two 7-segment
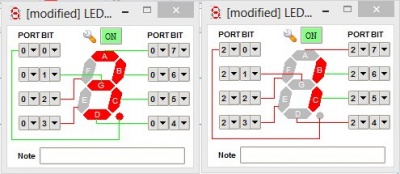
MOV DPTR,#TAB
tens: MOV P0,#00000010B
MOV R5,#01H
units: MOV R3,#00H
next: MOV A,R3
MOVC A,@A+DPTR
MOV P2,A
ACALL delay
INC R3
CJNE R3,#10D,next
MOV A,R5
MOVC A,@a+dptr
MOV P0,A
INC R5
CJNE R5,#11D,again
SJMP tens
again: SJMP units
delay: MOV R2,#06D
temp2: MOV R1,#255D
temp1: MOV R0,#255D
DJNZ R0,$
DJNZ R1,temp1
DJNZ R2,temp2
RET
TAB: DB 00000010B ;0x02 0D
DB 10011111B ;0x9F 1D
DB 00100100B ;0x24 2D
DB 00001100B ;0x1C 3D
DB 10011000B ;0x98 4D
DB 01001000B ;0x48 5D
DB 01000000B ;0x40 6D
DB 00011110B ;0x1E 7D
DB 00000000B ;0x00 8D
DB 00001000B ;0x0F 9D
END
Counter
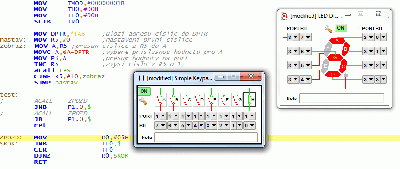
MOV TMOD,#00000001B
MOV TH0,#00H
MOV TL0,#00H
SETB TR0
MOV DPTR,#TAB ;
nastav: MOV R5,#0 ;
zobraz: MOV A,R5
MOVC A,@A+DPTR ;
MOV P3,A
INC R5 ;
acall test
CJNE R5,#10,zobraz
SJMP nastav
test:
; ACALL ZPOZD
JNB P1.0,$ ;
; ACALL ZPOZD
JB P1.0,$ ;
ret
ZPOZD: MOV R0,#05H
SKOK: JNB TF0,$
CLR TF0
DJNZ R0,SKOK
RET
TAB: DB 0C0h,0F9H,0A4H,0B0H,99H ;0,1,2,3,4,
DB 92H,82H,0F8H,80H,90H ;5,6,7,8,9,
end
L293
L293D_A equ P2.0 ;L293D A - Positive of Motor
L293D_B equ P2.1 ;L293D B - Negative of Motor
L293D_E equ P2.2 ;L293D E - Enable pin of IC
org 0H
Main:
acall rotate_f ;Rotate motor forward
acall delay ;Let the motor rotate
acall break ;Stop the motor
acall delay ;Wait for some time
acall rotate_b ;Rotate motor backward
acall delay ;Let the motor rotate
acall break ;Stop the motor
acall delay ;Wait for some time
sjmp Main ;Do this in loop
rotate_f:
setb L293D_A ;Make Positive of motor 1
clr L293D_B ;Make negative of motor 0
setb L293D_E ;Enable to run the motor
ret ;Return from routine
rotate_b:
clr L293D_A ;Make positive of motor 0
setb L293D_B ;Make negative of motor 1
setb L293D_E ;Enable to run the motor
ret ;Return from routine
break:
clr L293D_A ;Make Positive of motor 0
clr L293D_B ;Make negative of motor 0
clr L293D_E ;Disable the o/p
ret ;Return from routine
delay: mov r7,#20H
back: mov r6,#FFH
back1: mov r5,#FFH
here: djnz r5, here
djnz r6, back1
djnz r7, back
ret
#include <at89x51.h>
#define L293D_A P2_0 //Positive of motor
#define L293D_B P2_1 //Negative of motor
#define L293D_E P2_2 //Enable of L293D
// Function Prototypes
void rotate_f(void); //Forward run funtion
void rotate_b(void); //Backward run function
void breaks(void); //Motor stop function
void delay(void); //Some delay
void main(){ //
while(1){ //
rotate_f(); //
delay(); //
breaks(); //
delay(); //
rotate_b(); //
delay(); //
breaks(); //
delay(); //
} //
}
void rotate_f(){
L293D_A = 1; //
L293D_B = 0; //
L293D_E = 1; //
}
void rotate_b(){
L293D_A = 0; //
L293D_B = 1; //
L293D_E = 1; //
}
void breaks(){
L293D_A = 0; //
L293D_B = 0; //
L293D_E = 0; //
}
void delay(){ //
unsigned char i,j,k;
for(i=0;i<32;i++)
for(j=0;j<255;j++)
for(k=0;k<255;k++);
}
ATM13
MOV DPTR,#TAB
tens: MOV P0,#00000010B
MOV R5,#01H
units: MOV R3,#00H
next: MOV A,R3
MOVC A,@A+DPTR
MOV P0,A
ACALL delay
INC R3
CJNE R3,#10D,next
MOV A,R5
MOVC A,@a+dptr
MOV P2,A
INC R5
CJNE R5,#11D,again
SJMP tens
again: SJMP units
delay: MOV R2,#06D
temp2: MOV R1,#255D
temp1: MOV R0,#255D
DJNZ R0,$
DJNZ R1,temp1
DJNZ R2,temp2
RET
TAB: DB 01000010B ;0x02 0D
DB 10011111B ;0x9F 1D
DB 00100100B ;0x24 2D
DB 00001100B ;0x1C 3D
DB 10011000B ;0x98 4D
DB 01001000B ;0x48 5D
DB 01000000B ;0x40 6D
DB 00011110B ;0x1E 7D
DB 00000000B ;0x00 8D
DB 00001000B ;0x0F 9D
END
//2 Digit 7-Segment Up Down Counter Project using 8051 Microcontroller
//https://electronicswork.wordpress.com/2012/10/10/2-digit-7-segment-up-down-counter-project-using-8051-microcontroller/
#include <at89x51.h>
#define h P3_2 //up counter button
#define g P3_3 //down counter button
int m=0;
int n=0;
int a,b;
int arr[10]={0x21,0x7D,0x13,0x19,0x4D,0x89,0x81,0x3D,0x01,0x09};
void main()
{
P2=0x21;
P0=0x21;
while(1)
{
P3=0xFF;
if(h==0)
{
if(n==99&&m==99)
{
P2=0xFE;
P0=0xFE;
}
else
{
m=m+1;
n=n+1;
a=m/10;
b=n%10;
P2=arr[a];
P0=arr[b];
while(h==0);
}
}
if(g==0)
{
if(n==0&&m==0)
{
P2=0x3F;
P0=0x3F;
}
else
{
m=m-1;
n=n-1;
a=m/10;
b=n%10;
P2=arr[a];
P0=arr[b];
while(g==0);
}
}
}
}
Jak na barevnou syntaxi do Wordu
-
Použít Export as XHTML
-
V prohlížeči CTRL-C
-
Do Wordu CTRL-V při zachování formátování